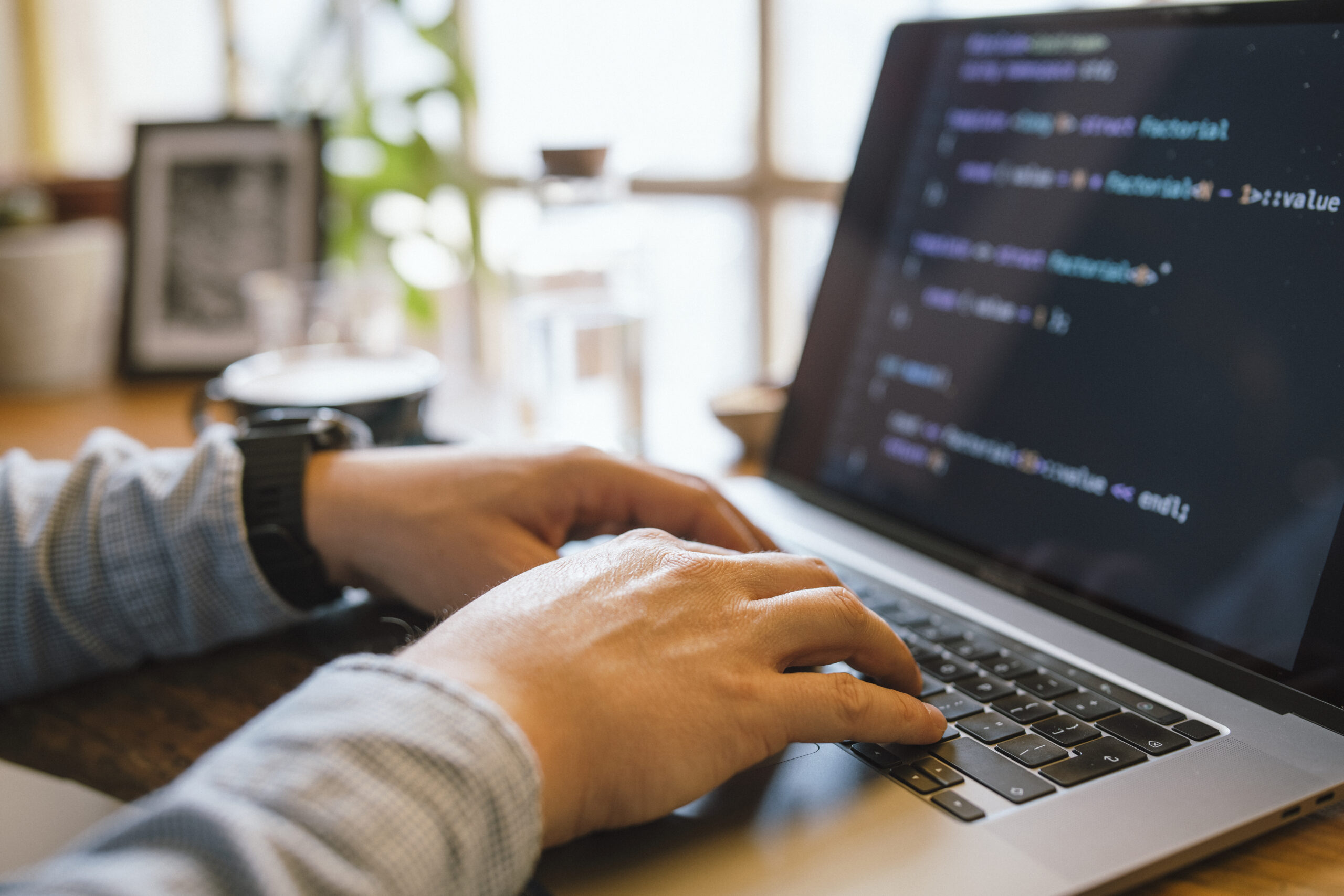
Debugging is Probably the most necessary — yet usually forgotten — skills inside a developer’s toolkit. It isn't nearly repairing broken code; it’s about knowledge how and why points go Completely wrong, and learning to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can preserve hours of disappointment and substantially boost your productiveness. Listed below are various tactics that can help developers degree up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though composing code is 1 part of improvement, knowing ways to communicate with it efficiently during execution is Similarly significant. Present day improvement environments appear equipped with potent debugging abilities — but a lot of builders only scratch the surface of what these applications can do.
Choose, by way of example, an Integrated Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools enable you to set breakpoints, inspect the worth of variables at runtime, step through code line by line, and in some cases modify code around the fly. When applied appropriately, they Permit you to observe precisely how your code behaves through execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check community requests, view true-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can transform irritating UI troubles into workable duties.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage around operating processes and memory management. Mastering these applications may have a steeper Understanding curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfy with Edition Management systems like Git to comprehend code historical past, come across the precise instant bugs were introduced, and isolate problematic improvements.
Finally, mastering your applications means going past default settings and shortcuts — it’s about creating an personal knowledge of your advancement setting to ensure when difficulties occur, you’re not missing in the dark. The better you know your tools, the greater time you could expend resolving the particular trouble rather then fumbling by the method.
Reproduce the challenge
The most significant — and infrequently neglected — methods in successful debugging is reproducing the trouble. Prior to leaping into the code or making guesses, developers require to produce a dependable setting or situation the place the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of chance, normally bringing about squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting just as much context as is possible. Inquire queries like: What steps brought about the issue? Which natural environment was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you have, the much easier it gets to be to isolate the precise situations less than which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If The problem seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions involved. These assessments not only aid expose the condition but additionally avert regressions Down the road.
From time to time, The difficulty might be setting-unique — it might take place only on selected functioning programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a phase — it’s a frame of mind. It necessitates tolerance, observation, and a methodical solution. But once you can regularly recreate the bug, you are previously midway to repairing it. By using a reproducible scenario, you can use your debugging resources much more efficiently, check prospective fixes securely, and talk additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s in which developers thrive.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most respected clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders must understand to deal with error messages as immediate communications through the program. They frequently show you what precisely transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading the information diligently and in complete. Lots of developers, especially when underneath time stress, look at the 1st line and quickly begin earning assumptions. But further in the mistake stack or logs might lie the legitimate root induce. Don’t just copy and paste mistake messages into serps — go through and understand them 1st.
Break the error down into pieces. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a particular file and line number? What module or operate induced it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context wherein the error transpired. Look at associated log entries, input values, and up to date improvements in the codebase.
Don’t neglect compiler or linter warnings both. These typically precede greater troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, serving to you pinpoint issues quicker, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most potent resources within a developer’s debugging toolkit. When employed properly, it provides actual-time insights into how an application behaves, aiding you realize what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
An excellent logging method begins with understanding what to log and at what stage. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for in depth diagnostic facts all through enhancement, Details for standard activities (like productive begin-ups), Alert for probable troubles that don’t crack the appliance, ERROR for precise troubles, and Deadly when the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant information. Too much logging can obscure critical messages and slow down your procedure. Target important events, condition modifications, input/output values, and important determination points as part of your code.
Format your log messages Evidently and constantly. Include context, such as timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping by code isn’t feasible.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-imagined-out logging method, you may lessen the time it will take to identify difficulties, acquire deeper visibility into your apps, and Increase the In general maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently detect and correct bugs, builders will have to method the process just like a detective fixing a thriller. This way of thinking helps break down intricate challenges into workable components and adhere to clues logically to uncover the basis lead to.
Start off by accumulating proof. Consider the signs or symptoms of the problem: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much relevant info as you can with out jumping to conclusions. Use logs, test instances, and user reports to piece together a transparent photograph of what’s going on.
Subsequent, form hypotheses. Inquire your self: What might be creating this behavior? Have any changes lately been made into the codebase? Has this concern transpired prior to under similar instances? The target is usually to slim down choices and identify opportunity culprits.
Then, test your theories systematically. Try to recreate the condition in the controlled ecosystem. When you suspect a particular operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay shut focus to little aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-just one error, or a race issue. Be thorough and individual, resisting the urge to patch The difficulty with no absolutely comprehension it. Temporary fixes could disguise the real trouble, only for it to resurface later on.
Lastly, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging course of action can save time for potential challenges and support others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed concerns in intricate units.
Write Exams
Composing assessments is among the simplest ways to enhance your debugging expertise and Total improvement efficiency. Exams not merely support capture bugs early and also function a security Web that offers you assurance when making adjustments to the codebase. A properly-examined software is simpler to debug since it permits you to pinpoint accurately where by and when a dilemma takes place.
Get started with device checks, which deal with individual capabilities or modules. These compact, isolated assessments can promptly expose no matter whether a certain piece of logic is Operating as anticipated. Whenever a check fails, you immediately know where to glimpse, noticeably cutting down enough time invested debugging. Unit checks are In particular valuable for catching regression bugs—difficulties that reappear soon after Formerly being preset.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of elements of your software operate collectively smoothly. They’re specially beneficial for catching bugs that take place in complex devices with several components or expert services interacting. If a thing breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a attribute effectively, you need to grasp its inputs, expected outputs, and edge situations. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing test that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to focus on fixing the bug and look at your exam pass when The problem is solved. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, more rapidly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your monitor for several hours, trying Answer right after Resolution. But one of the most underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease aggravation, and often see the issue from a new standpoint.
If you're much too near the code for as well lengthy, cognitive fatigue sets in. You may start overlooking obvious errors or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be less economical at issue-solving. A brief stroll, a coffee crack, or maybe switching to a unique process for ten–15 minutes can refresh your focus. Many builders report obtaining the root of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, especially all through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You may instantly observe a read more missing semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re stuck, a very good guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath tight deadlines, but it surely really brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks is just not an indication of weakness—it’s a wise tactic. It gives your brain Room to breathe, increases your point of view, and allows you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It really is a chance to improve as a developer. Regardless of whether it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, every one can teach you some thing useful when you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking you a handful of key questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit testing, code critiques, or logging? The answers frequently reveal blind places in your workflow or understanding and enable you to Construct more powerful coding routines shifting ahead.
Documenting bugs will also be a wonderful practice. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring concerns or prevalent problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically potent. Whether it’s by way of a Slack message, a brief publish-up, or a quick know-how-sharing session, supporting Some others stay away from the same challenge boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. All things considered, a few of the finest developers are not the ones who generate excellent code, but those who continually master from their blunders.
Eventually, Each and every bug you deal with adds a whole new layer towards your skill set. So future time you squash a bug, take a second to mirror—you’ll occur away a smarter, far more capable developer on account of it.
Summary
Improving your debugging capabilities usually takes time, practice, and persistence — although the payoff is large. It will make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.